top of page
Project Brief:
Make a clock with p5js. Functions like second(), hour() and minute() are useful here! The clock can be analogous to a real-world clock or it could be an abstract way of telling time
Inspired by The Coding Train video Coding Challenge #74: Clock with p5.js.
https://www.youtube.com/watch?v=E4RyStef-gY&t=1010s&ab_channel=TheCodingTrain
I decided to use a different color of the stroke to create ellipses in order to represent seconds, minutes, and hours.

let hr = hour();
let mn = minute();
let sc = second();
//second outline
push();
strokeWeight(10);
stroke(120, sc + 30, sc * 5);
// fill(120, sc * 2, 30);
let scAngle = map(sc, 0, 60, 0, 360);
arc(0, 0, 260, 260, 0, scAngle);
pop();
//minute outline
strokeWeight(10);
stroke(300, mn * 20, 75);
noFill();
let mnAngle = map(mn, 0, 60, 0, 360);
arc(0, 0, 280, 280, 0, mnAngle);
//hour outline
strokeWeight(10),
stroke(200, 54, 70);
noFill();
let hrAngle = map(hr % 12, 0, 12, 0, 360);
arc(0, 0, 300, 300, 0, hrAngle);
//* not a full code
Use map() to set the data of current time, then use it to draw arc. For the second outline, I decided to change the fill color base on the second added.
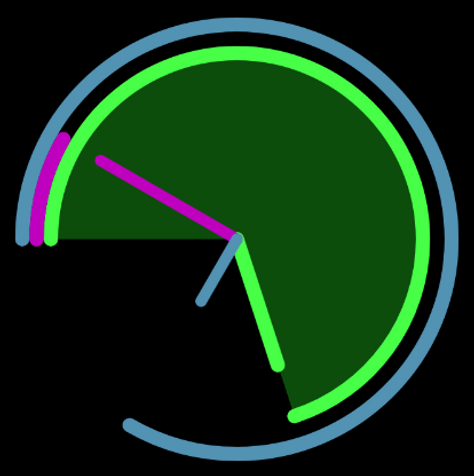
//second pointer
push();
let i = 1
while(i < sc){
i = sc * 2.2
}
stroke(120, sc + 30, sc * 5);
rotate(scAngle);
line(0, 0, i, 0);
pop()
Adding pointer in matching color. For the second point, I use a while loop to let it grow based on the second. And also, the background fill by the second pointer is changeable base on the second.

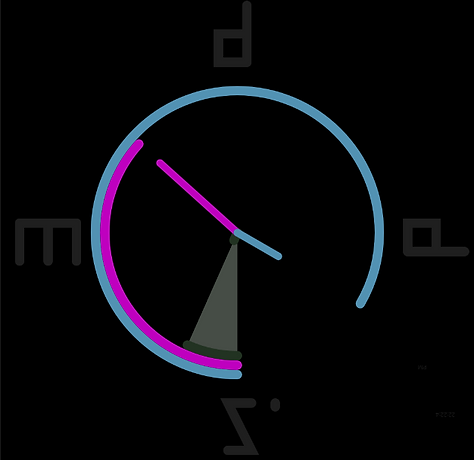
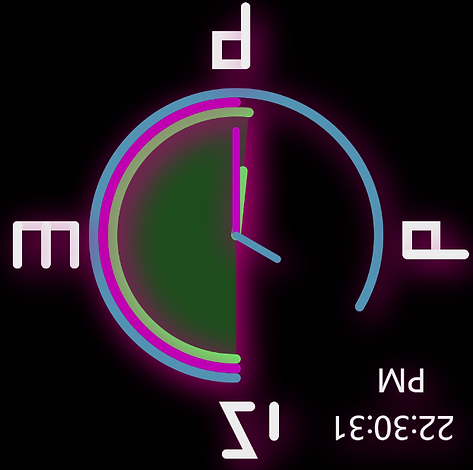
Adding numbers on each side of the clock. I make the numbers all reversed on purpose. That was inspired by a dream study I did for my VR class. During that specific dream I had, I was in a world where everything is reversed, upside down. So I want to create a clock from that world.
The number "1" is growing based on seconds by using the if statement.
let twlX = 180
if(twlX < 220){
twlX = 180 + sc
line(180, -40, twlX, -40)
if(twlX = 230){
return
}
Also, the fill of each number is depending on the seconds.
stroke(sc * 3);
Adding 24hr time in the text on the bottom right. Also growing depending on second. Using if statement to detect if it's AM or PM.
textSize(sc + 2)
fill(sc * 3);
text(hr + ':'+ mn + ':' + sc, -230, -190);
let noon = hr
if(hr > 12){
text('PM', -200, -140)
}
else{
text('AM', -200, -140)
}
Finally, using drawingContext to add a faded shadow on the back to give a cyberpunk feeling.
let offsetX = map(sc * 5, 0, width, sc, -20);
let offsetY = map(sc * 5, 0, height, sc, -20);
drawingContext.shadowOffsetX = offsetX;
drawingContext.shadowOffsetY = offsetY;
drawingContext.shadowBlur = 66;
drawingContext.shadowColor = color(320, 100, 76);
Project display
bottom of page