top of page
Project display
Project Brief:
Create a sketch that calls an API, processes the data, and visualizes it somehow. A non-exhaistive list of publicly available APIs can be found here
After learning from class and watching videoes about API & Json from The Coding Train, I decided to create a mini weather report app. I'm trying to make it easy to visualize, but also contain all information you need.

Starting with creating the background for the "APP". I decided to create 3 rectangles to put data. On the top left, I decided to put in UI icon for different weather conditions on the top left. I believe simple UI can visually show information user needs.
On the bottom left I decide to add UI icons of my advise for the specific weather.
On the right side, I decide to print out all the important information that the user needs.
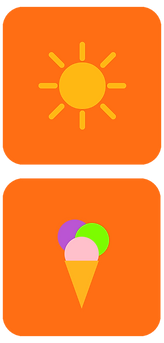
Sun icon with ice cream for clear weather and temperature over 20 °C

Sun icon with sun glasses for clear weather and temperature under 15 °C

Cloudy icon for cloudy/haze/mist weather condition and coffee icon as my advise. (You need coffee in that weather!
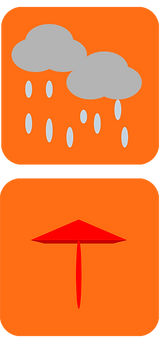
Raining icon and umbrella for raining weather.

Snow icon with snowman for snow weather.
Then I got data from api.openweathermap.org/data/2.5/weather.
let api = 'https://api.openweathermap.org/data/2.5/weather?q=';
let apiKey = ?
let units = '&units=metric';
let input = 'New York';
function searchWeather(){
let url = api + input.value() + apiKey + units
loadJSON(url, gotData);
}
function gotData(data){
weather = data;
console.log(data.weather[0].main)
}
Setting up button and search part on top for user input
</body>
<p>
City: <input id = "city" value="New York"></input>
<button id="search">search</button>
</p>
</html>
let button = select('#search')
button.mousePressed(searchWeather)
input = select('#city')
After getting the data, I start to call out functions for those icons based on the weather conditions/temperature by if() statement. Also, The background color is also affected by the temperature. I put all these conditions into one function. Specifically for rain & snow conditions, I created animation for them. Last but not list, add a gif as background.
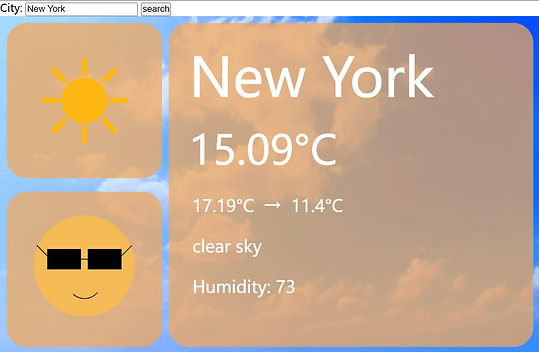

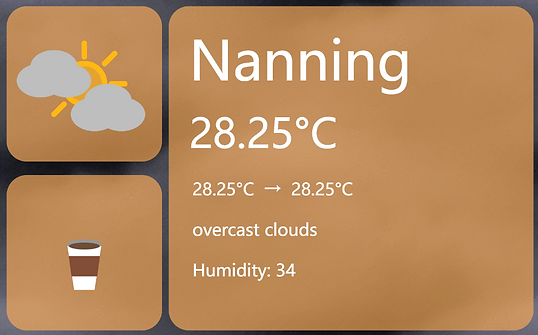

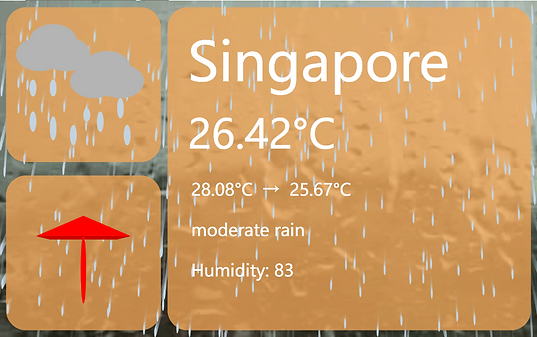

function weatherConditions(){
// ----- fill different colors for different temperature
if(weather.main.temp > 15){
fill(245, 167, 87, 90)
}if(weather.main.temp > 30){
fill(255, 90, 0)
}if(weather.main.temp < 15){
fill(118,132,220)
}if(weather.main.temp < 0){
fill(65,93,177)
}
// ----- get data for weather conditions and use specific icons to indicate them
if(weather.weather[0].main=='Rain'){
// ----- load gif for rain background
image(gif_loadRainimg, 0, 0, 800, 500)
tint(255, 25)
// ----- bring up raining animation
for (let i = 0; i < rainArray.length; i++) {
rainArray[i].raining()
}
rainingIcon();
umbrella();
printCityinfo();
}
if(weather.weather[0].main=='Thunderstorm'){
// ----- load background thunder gif
image(gif_loadThunderimg, 0, 0, 800, 500)
tint(255, 35)
// ----- bring up raining animation
for (let i = 0; i < rainArray.length; i++) {
rainArray[i].raining()
}
thunderStorm();
umbrella();
printCityinfo();
}
if(weather.weather[0].main=='Clear'){
// fill(255, 187, 87, 100)
// ----- load background clear day gif
image(gif_loadSunnyimg, 0, 0, 800, 500)
tint(255, 30)
sunIcon();
// ----- if temperature >20, my advise is ice cream! Else it would be put on sun glasses
if(weather.main.temp > 20){
iceCream();}
else{
sunGlasses();
}
printCityinfo();
}
if(weather.weather[0].main=='Clouds'){
// fill(255, 187, 87, 100)
// ----- load background cloud img
image(gif_loadCloudimg, 0, 0, 800, 500)
tint(255, 35)
cloudyIcon();
coffee();
// console.log(weather)
printCityinfo();
}
if(weather.weather[0].main=='Smoke'){
// fill(255, 187, 87, 100)
// ----- load background cloud img
image(gif_loadCloudimg, 0, 0, 800, 500)
tint(255, 35)
cloudyIcon();
coffee();
// console.log(weather)
printCityinfo();
}
if(weather.weather[0].main=='Haze'){
image(gif_loadCloudimg, 0, 0, 800, 500)
tint(255, 35)
hazeIcon();
coffee();
printCityinfo();
}
if(weather.weather[0].main=='Mist'){
image(gif_loadCloudimg, 0, 0, 800, 500)
tint(255, 35)
hazeIcon();
coffee();
printCityinfo();
}
if(weather.weather[0].main=='Drizzle'){
image(gif_loadCloudimg, 0, 0, 800, 500)
tint(255, 35)
hazeIcon();
coffee();
printCityinfo();
}
if(weather.weather[0].main=='Snow'){
image(img_loadSnowimg, 0, 0, 800, 500)
tint(255, 35)
for (let g = 0; g < snowArray.length; g++) {
snowArray[g].snowing()
}
snowMan();
snowIcon();
printCityinfo();
}
}
In the end, I don't want my mini-app to start with a blank surface and only the search button. I use the if( ) statement the preset the URL to New York(where I live in). So that the app will start with displaying New York's current weather.
let button = select('#search')
let url = api + 'New York' + apiKey + units
loadJSON(url, gotData)
if(button.mousePressed){
button.mousePressed(searchWeather)
input = select('#city')
}
bottom of page